JavaScript is a programming language that is only available in web browsers. The JavaScript console is a command-line interface in your browser that allows you to run code snippets. When that code snippet is designed to interact with the webpage you are currently viewing, unexpected results may occur.
“PrintIn” makes the text be printed to the console, whereas you may use “console.log” to log it and use it for various purposes, such as emailing it as a bug report.
If you’re a programmer, you know how important to use and understand scripting languages like JavaScript is. However, there are times that you may be confused about its functions.
Just like the printIn and console.log function. To help you understand the difference and application of these two functions, I’ll explain what they mean and how they work.
Let’s begin!
What is JavaScript?
JavaScript is a scripting language for creating regularly updated material, controlling multimedia, animating graphics, and pretty much anything else.
The JavaScript programming language has certain standard programming capabilities that enable you to do things like:
- When certain events occur on a web page, you can respond to a running code.
- You can use variables to store useful data.
- You can use “strings” which is a text editing operation in programming
The functionality added on top of the user JavaScript language, on the other hand, is even more interesting. Application Programming Interfaces (APIs) give your JavaScript code extra functions.
In short, JavaScript has a lot of functions that allow you to control what you are coding. These functions include printIn and console.log.
What is PrintIn?
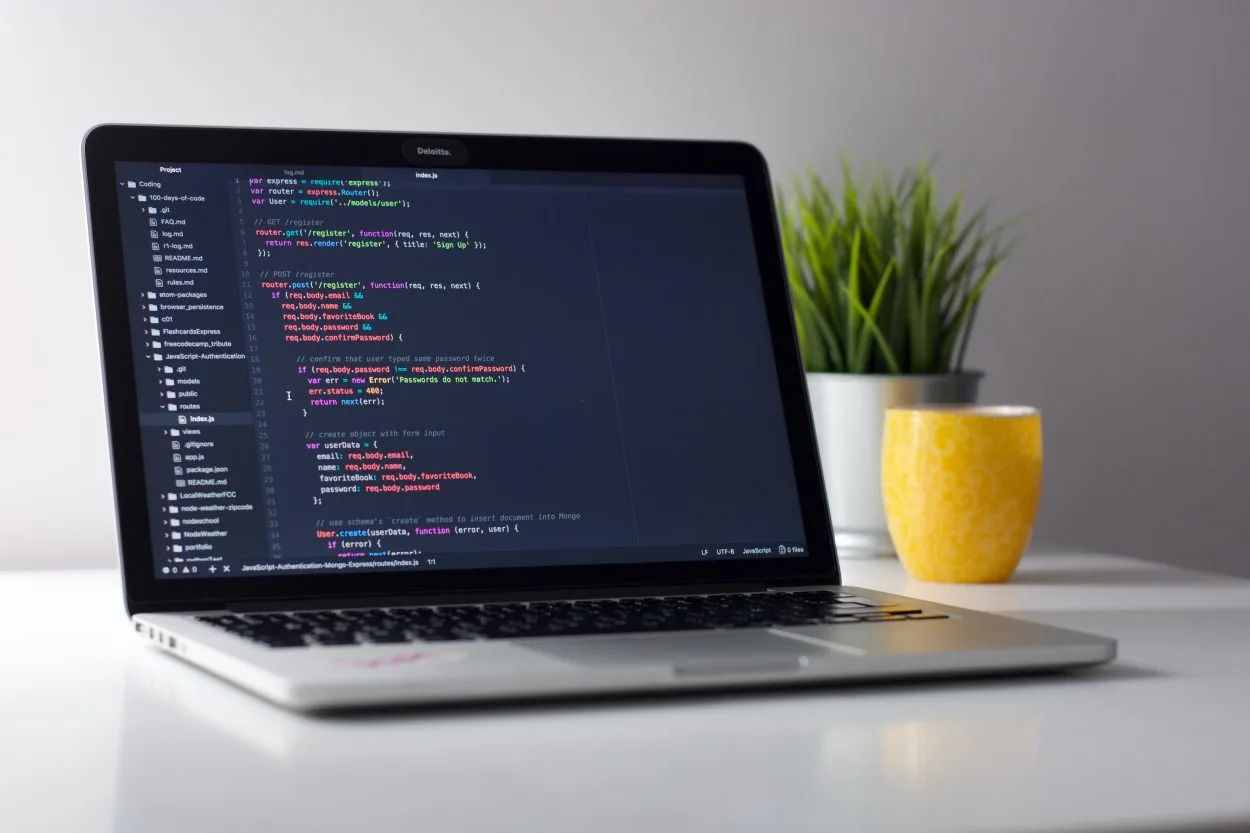
PrintIn is a Java method for displaying text on the console. This method accepts this text as a parameter in the form of a String. This approach prints the text to the console while keeping the cursor at the start of the following line.
The next printing begins on the next line. There are several printIn methods like:
void printIn() | Writes the line separator string to end the current line. |
void printIn(boolean x) | The line is terminated after printing a boolean. |
void printIn(char x) | The line is terminated after printing a character. |
void print(char [ ] x) | The line is terminated after printing an array of characters. |
void printIn(double x) | The line is terminated after printing a double line. |
void printIn(float x) | The line is terminated after printing afloat. |
void printIn(int x) | The line is terminated after printing an integer. |
void printIn(long x) | The line is terminated after printing along. |
void printIn(Object x) | The line is terminated after printing an object. |
void printIn(String x) | The line is terminated after printing a string. |
Although it has many methods that you may use in coding your work, you may encounter another method for displaying the text in the console. In the console, there are two methods where you can print your work, the first one is printIn while the other one is print.
For you to be able to not get confused between these two methods of printing, let’s define the difference between the second method in printing, the print.
Print is a Java method for displaying text on the console. This method accepts this text as a parameter in the form of a String. This approach prints the text to the console while keeping the cursor at the end of the following line.
The next printing will begin right here. There are several printIn methods like:
void print(boolean b) | A boolean value is printed. |
void print(char c) | A character is printed. |
void print(char [ ] s) | An array of characters are printed. |
void print(double d) | A double-precision floating-point number is printed. |
void print(float f) | A floating-point number is printed. |
void print(int i) | An integer is printed. |
void print(long l) | A long integer is printed. |
void print(Object obj) | An object is printed. |
void print(String s) | A string is printed. |
In short, the key difference between the two is the placement of the text printed in the console. PrintIn is at the start of the following line while Print is at the end of the following line.
If you’re interested to know about windows 10-pro and pro-n, check out my other article.
What is Console.log?
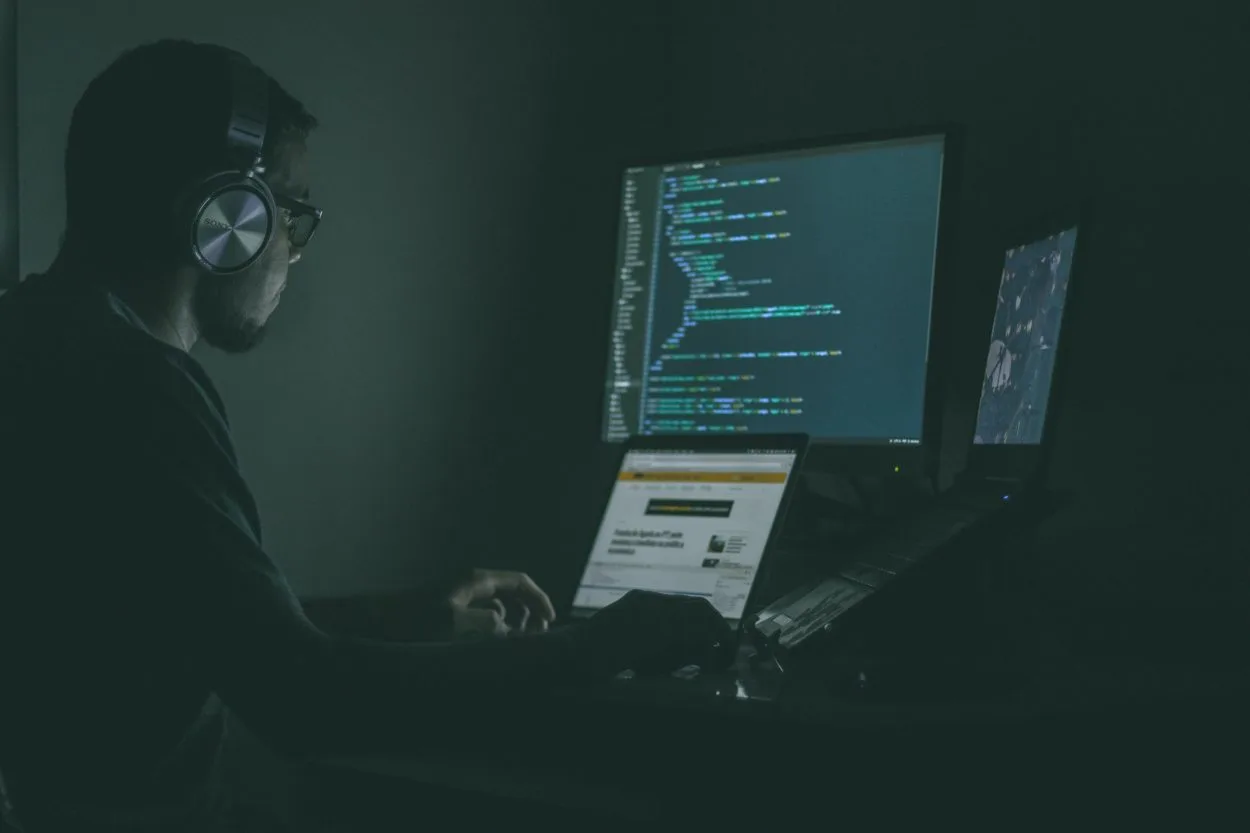
The console is a JavaScript object that gives you access to the browser’s debugging console.
The console.log is a JavaScript function that prints any variables that have been defined previously in it, as well as any information that needs to be shown to the user.
The output is mostly logged (printed) to the terminal. Any type can be passed to log(), including strings, arrays, objects, and booleans.
The console.log() method’s output is visible in the JavaScript console, which is accessible via the browser’s developer tool. Whatever you output with console.log() is accessible to all end-users, regardless of their group or role.
Let’s take a look at how you can use it, and the output after using this function.
JavaScript | Output |
// console. log() methodconsole.log('abc'); console.log(1);
g g | abc 1 true null undefined Array(4) [ 1, 2, 3, 4 ] Object { a : 1, b : 2 , c : 3 } |
What is the Print to Console with Console.log method in Javascript?
It is JavaScript’s most popular and widely used console method. This method is frequently used to print various messages or calculation results to the console or even while debugging code.
You’ve written some code that adds two numbers, and you’d like to see the result of that operation on the console; in this case, you can use the console.log() method.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<script>
var num_1 = 2, num_2 = 3;
console.log(num_1 + num_2);
</script>
</body>
</html>
Is Console.log synchronous or asynchronous?
Before I discuss with you whether console.log is synchronous or asynchronous, I’ll define first what synchronous and asynchronous are.
Synchronous means that it occurs at the same time whereas asynchronous means that it does not occur at the same time. So participants/users in synchronous can receive feedback immediately. Asynchronous allows you to learn in your own time.
To answer, concole.log is asynchronous. I’ll show you why is that, by showing examples, particularly sorting an array of objects in a more efficient manner. Let’s start.
Let’s say you have the following objects in your array:
let users = [ { name: “Nicole” , age: 20, surname: “Luna” } , { name: “Cara” , age: 21, surname: “Lim” } , { name: “Lara” , age: 20, surname: “Tuazon” }; ] |
You must sort this array by field name, which is typically done as follows.
/ / by name ( Cara, Lara, Nicole ) users.sort ( ( a, b ) => a.name > b.name ? 1 : -1); / / by age ( Lara, Nicole, Cara ) users.sort ( ( a, b ) => a.age > b.name ? 1 : -1); |
To arrange it in more efficient manner, you’ll have like this:
users.sort(byField( ‘name’ )); users.sort(byField( ‘age’ )); |
To do so, you must write the “Byfield” function to pass and sort it to Array.prototype.sort of the objects in your array. Well, this is not the primary focus of this article but please look below example to complete the above example in a simple way.
> let users = [ { name: “Nicole” , age: 20, surname: “Luna” } , { name: “Cara” , age: 21, surname: “Lim” } , { name: “Lara” , age: 20, surname: “Tuazon” }; ] function byField ( fieldName ){ return (a, b ) => a[fieldName] ? 1 : -1 ; } users.sort(byField( ‘name’ ) ); concole.log(users); users.sort(byField( ‘age’ ) ); concole.log(users); (3) [ { … }, { … }, { … } ] > 0: { name: ” Lara “, age: ” 20 ” , surname: ” Tuazon ” } > 1: { name: ” Nicole “, age: ” 20 ” , surname: ” Luna ” } > 1: { name: ” Cara “, age: ” 21 ” , surname: ” Lim ” } length: 3 > _proto_: Array (0) (3) [ { … }, { … }, { … } ] > 0: { name: ” Lara “, age: ” 20 ” , surname: ” Tuazon ” } > 1: { name: ” Nicole “, age: ” 20 ” , surname: ” Luna ” } > 1: { name: ” Cara “, age: ” 21 ” , surname: ” Lim ” } length: 3 > _proto_: Array (0) |
You can see from the table above that I sort the array objects twice, I sort by name first, next by age, and after each sort operation, I run console.log (). Also, you may have observed that console.log() restored the same output for every sort of result, but this is not the case; let me explain why.
I ran the code above all at once, then decided to expand every reaction from console.log (). This is significant because console.log() is asynchronous.
In terms of Event Loop, all asynchronous features arrive at the Event Table. In this case, after bringing up console.log(), it proceeds to Event Table and waits for a specific event to occur.
When an event occurs, console.log() will be sent to Event Queue, where it waits until all processes in this Event Queue that were present already when your console.log is placed and have been sent to Call Stack, then your console.log() is being sent to this Call Stack as well.
How to Open the Javascript Console.log?
In web browsers, the Console is one of the several Developer Tools. In order to troubleshoot your JavaScript code, you can use the Console. The Console can be found in many places depending on the browser.
I’ll teach you where to discover the Console in your Google Chrome browser in this tutorial.
Steps on how to open the console log in Chrome
Let’s look at how to open the Chrome console log.
- Select Inspect from the pop-up menu while the Chrome browser is open.
- The Developer Tools’ “Elements” tab will be opened by default when you run Inspect. To the right of “Elements,” click “Console.”
- You may now view the Console as well as any output recorded to the Console log.
You may also open the Chrome Developer Tools using a number of shortcut keys. According to the version of your Chrome, you can use the following shortcuts:
For Windows and Linux,
Ctrl + Shift + I | The Developer Tools window appears. |
Ctrl + Shift + J | Selects the Console tab in the Developer Tools. |
Ctrl + Shift + C | Inspect Element mode toggles |
Final Thoughts
The main difference between printIn and console.log is their function and the outcome of the code. PrintIn prints the text to the console while console.log prints any variables with strings that have been coded before.
Basically, these functions of Javascript allow you to print and display the variables and text to the console. In JavaScript, you can print using a variety of methods.
The JavaScript console log method is the most commonly used option when debugging. To debug your code more effectively, you should practice them all and learn how to use them correctly.
Programmers and developers often used these to print any variables that have been predefined in it, as well as any information that needs to be presented to the user.